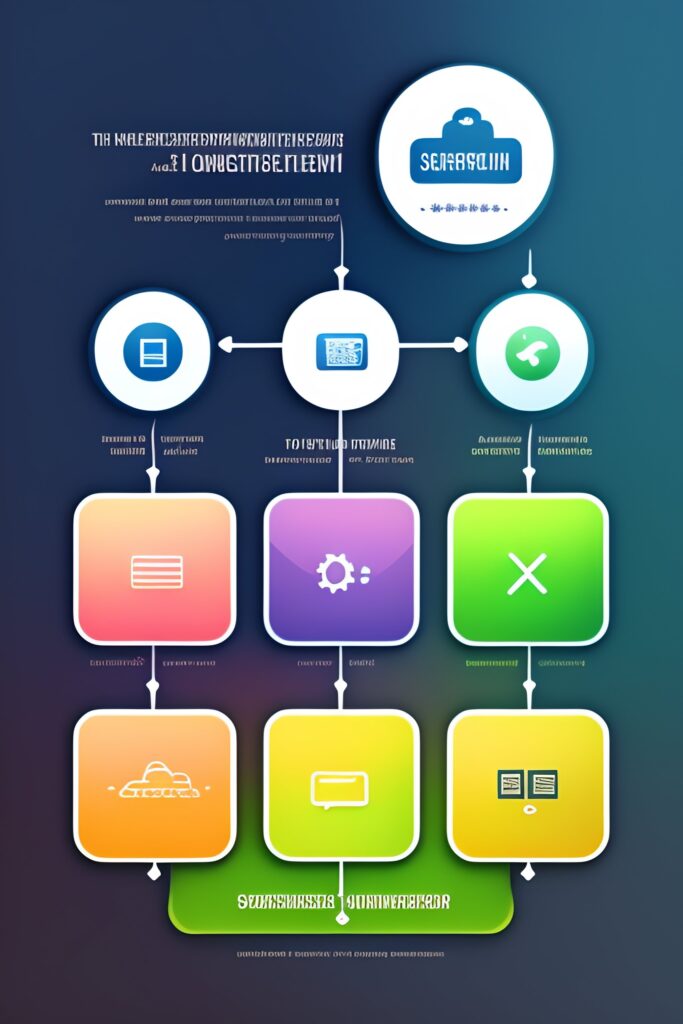
Source Code Process:
Grant merging access only to Team Leads (TLs) and not all developers. This ensures that only those who are responsible for merging code have the ability to do so. This helps to prevent unauthorized changes from being made to the codebase.
Perform unit testing before submitting a code merge request. This ensures that the code works as expected before it is merged into the main codebase. This helps to prevent errors from being introduced into the codebase.
Ensure that code review is conducted before merging any code merge request. This is an important step in ensuring that the code is of high quality and that it meets the requirements of the project. This helps to prevent bugs from being introduced into the codebase.
Remove access in a timely manner for individuals who no longer require it. This helps to protect the security of the codebase and to prevent unauthorized access.
Validate the business logic and assess the impact during code review. This ensures that the code changes make sense from a business perspective and that they do not have any unintended consequences.
Prepare a supporting document or update the solutioning document with actions and checkpoints related to the code changes. This helps to keep track of the changes that have been made to the codebase and to ensure that they are properly documented.
When code is merging on PROD that time Live API details and other credentias must be validated. This ensures that the code changes do not expose sensitive information to unauthorized users.
During code review, table related changes must be highlighted and putted in a checklist. This helps to ensure that all of the changes to tables are properly reviewed and that no errors are introduced.
Unit test / QA must validate that the changes must fullfilled / completed the requirements and points listed in BRD/PRD. This ensures that the code changes meet the requirements of the project and that they do not introduce any new bugs.

Source Code Management Best Practices:
Regularly Commit Changes: Make frequent commits to the repository to track progress and maintain a history of changes. Avoid making large, monolithic commits and instead break them down into smaller, logical units.
Always Ensure That the Repository is Up to Date: Before starting any work, pull the latest changes from the remote repository to ensure you are working with the most recent code. This helps prevent conflicts and keeps your local copy in sync with the team.
Create Detailed Commit Messages: Provide clear and descriptive commit messages that explain the purpose and impact of the changes. This helps other team members understand the context and facilitates easier code reviews.
Review Changes Before Committing: Before committing your changes, review them carefully to ensure they adhere to coding standards, meet project requirements, and do not introduce any issues. This can be done through code reviews, pair programming, or automated testing.
Implement a Proper Workflow: Adopting a suitable version control workflow is crucial for maintaining an organized and efficient development process. I have written another post for the same and link is as follows:
http://techiefuel.prafulkr.info/git-branching-strategies/
Implement Proper Security Practices: We will have to protect our source code. So will have to implement security measures such as access controls, strong authentication, and encryption. Someone needs to monitor and audit access on a regular basis to the repository so an Authorized person can detect any unauthorized activity.

SAMPLE 1:
Initial Process Document for Git Workflow
Version: 1.0
Date: 07 July, 2023
Introduction:
This document outlines the initial process for managing source code using Git version control. It serves as a guide for developers, ensuring a standardized and efficient workflow throughout the development lifecycle.
Purpose:
The purpose of this document is to establish clear guidelines and best practices for using Git, enabling effective collaboration, code management, and version control within our development team.
Branching Strategy:
Select an appropriate branching strategy that aligns with our development methodology and project requirements. Consider options such as Git Flow, GitHub Flow, Trunk Based Development, or GitLab Flow. Evaluate the pros and cons of each strategy and choose the one that best suits our needs.
Repository Setup:
Set up a central Git repository to store the project’s source code. Ensure that the repository is accessible to all team members and properly configured with necessary access controls.
Branch Management:
Define guidelines for creating and managing branches:
- Create a development branch (e.g., “develop”) as the main branch for ongoing development.
- Create feature branches for new features or bug fixes, following a consistent naming convention.
- Merge feature branches into the development branch after code review and testing.
- Establish a release branch for each production release, merging stable code from the development branch.
- Maintain a stable “master” branch for production-ready code only.
Code Review:
Implement a code review process to ensure code quality and adherence to coding standards. Assign reviewers and establish clear criteria for approving code changes before merging them into the main branches.
Continuous Integration and Deployment:
Integrate Git with our CI/CD pipeline to enable automated builds, testing, and deployment. Configure triggers to initiate build processes upon successful code merges.
Collaboration Tools:
Utilize collaboration tools such as Git hosting platforms, issue trackers, and project management systems to enhance team collaboration, track progress, and manage tasks related to Git workflows.
Documentation:
Emphasize the importance of documenting changes, including commit messages, code comments, and any necessary supporting documentation. This promotes transparency and helps with future code maintenance and troubleshooting.
Training and Support:
Provide training and support to team members on Git fundamentals, branching strategies, and best practices. Encourage knowledge sharing and continuous improvement within the team.
Version Control Guidelines:
Establish guidelines for version control, including rules for tagging releases, managing version numbers, and handling hotfixes or critical patches.
Maintenance and Updates:
Regularly review and update this process document to reflect any changes in the project’s requirements or evolving best practices in Git workflows.
Please review this initial process document and provide any feedback, questions, or suggestions for improvement. Together, we can establish an effective Git workflow that enhances collaboration, code management, and overall development efficiency.
[Insert team members’ names and signatures for acknowledgment]
Note: This document will be subject to revisions and updates as the project progresses and requirements change.
SAMPLE 2:
Document Name: GIT-Initial Process Document
Version: 1.0
Date: 2023-07-07
- Objective
The objective of this document is to define the initial process for using Git for software development. This document will cover the following topics:
- Setting up a Git repository
- Creating and managing branches
- Committing and pushing changes
- Merging branches
- Rebasing branches
- Troubleshooting
- Setting up a Git repository
To set up a Git repository, you will need to install the Git software on your computer. Once you have installed Git, you can create a new repository by running the following command:
git init
This will create a new directory called .git in the current directory. The .git directory contains all of the information that Git needs to track your changes.
- Creating and managing branches
In Git, branches are used to track different versions of your code. To create a new branch, you can run the following command:
git checkout -b
For example, to create a new branch called feature-branch, you would run the following command
git checkout -b feature-branch
Once you have created a new branch, you can start making changes to your code. When you are finished making changes, you can commit your changes to the branch.
- Committing and pushing changes
To commit your changes to a branch, you can run the following command:
git commit -am “Commit message”
The -am flag tells Git to automatically add all of the modified files to the commit. The Commit message is a brief description of the changes that you have made.
Once you have committed your changes, you can push them to a remote repository. A remote repository is a Git repository that is stored on a server. To push your changes to a remote repository, you can run the following command:
git push origin
For example, to push your changes to a remote repository called origin and a branch called feature-branch, you would run the following command:
git push origin feature-branch
- Merging branches
Once you have finished working on a branch, you can merge it back into the main branch. To merge a branch, you can run the following command:
git merge
For example, to merge the feature-branch back into the main branch, you would run the following command:
git merge feature-branch
- Rebasing branches
Rebasing is a way to change the history of your branches. To rebase a branch, you can run the following command:
git rebase
For example, to rebase the feature-branch onto the main branch, you would run the following command:
git rebase main feature-branch
- Troubleshooting
If you encounter any problems with Git, you can consult the Git documentation: https://git-scm.com/docs/. You can also search for help online.
- Conclusion
This document has outlined the initial process for using Git for software development. This document should provide you with the basic knowledge that you need to get started with Git. For more information, please consult the Git documentation: https://git-scm.com/docs/.